Visual Studio 2019 v16.6より.NET CoreプロジェクトでのCOM参照の仕組みが追加されており、Visual Studio 2019 v16.8/.NET 5でもその機能を使うことが出来ます。こういうめんどくさいことはツールサポートが無いとやっていられません。
ここではコンソールアプリからExcelのCOM Automationを使う方法をサンプルとして提示したいと思います。
まず、Visual Studio 2019で.NET 5のコンソールアプリケーションのプロジェクトを作成し、以下のように依存関係のコンテキストメニューから「COM参照の追加」を選択します。
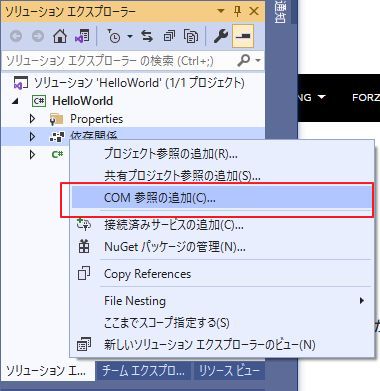
そうすると、見慣れたCOM参照の追加ダイアログが表示されます。
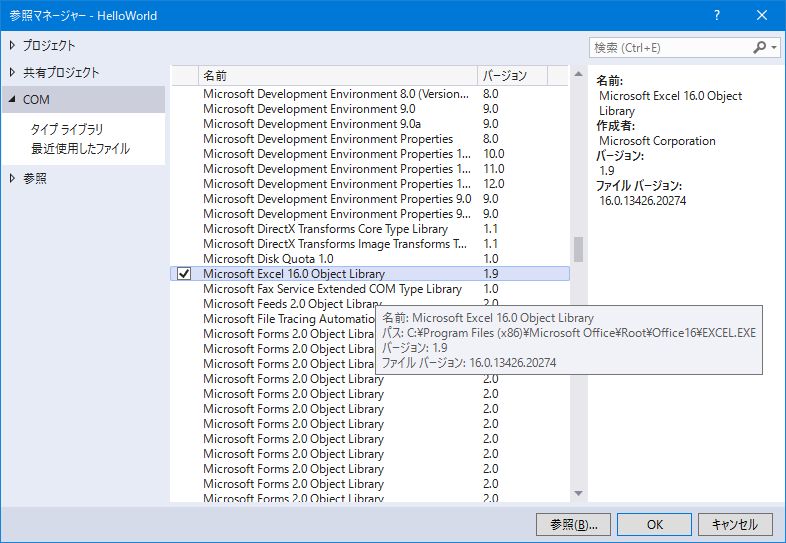
Excelを参照します。そうすると以下のようにinteropアセンブリが追加されます。

ここで大事なのは、このinteropアセンブリを選択して以下のように「ローカルコピー」「相互運用性の埋め込み」の両方を「はい」に設定しておきます。こうしておかないと実行時にSystem.IO.FileNotFoundException
のエラーになります。

必要な準備はこれだけです。これでcsprojファイルに以下のようにCOM参照の定義が追加され、必要なinteropアセンブリがプロジェクトに追加されます。
<Project Sdk="Microsoft.NET.Sdk"> <PropertyGroup> <OutputType>Exe</OutputType> <TargetFramework>net5.0</TargetFramework> </PropertyGroup> <PropertyGroup Condition="'$(Configuration)|$(Platform)'=='Debug|AnyCPU'"> <PlatformTarget>x86</PlatformTarget> </PropertyGroup> <ItemGroup> <COMReference Include="Microsoft.Office.Excel.dll"> <WrapperTool>tlbimp</WrapperTool> <VersionMinor>9</VersionMinor> <VersionMajor>1</VersionMajor> <Guid>00020813-0000-0000-c000-000000000046</Guid> <Lcid>0</Lcid> <Isolated>false</Isolated> <Private>true</Private> <EmbedInteropTypes>true</EmbedInteropTypes> </COMReference> </ItemGroup> </Project>
実際にExcelを使うコードは以下のようになりますが、これは.NET Frameworkの時と違いはありません。
using System; using System.Runtime.InteropServices; using Excel = Microsoft.Office.Interop.Excel; namespace HelloWorld { class Program { static void Main(string[] args) { var excelName = @"C:\temp\sample.xls"; Console.WriteLine("Hello World!"); var oXls = new Excel.Application(); oXls.Visible = true; var oWorkbook = oXls.Workbooks.Open( excelName, // オープンするExcelファイル名 Type.Missing, // (省略可能)UpdateLinks (0 / 1 / 2 / 3) Type.Missing, // (省略可能)ReadOnly (True / False ) Type.Missing, // (省略可能)Format // 1:タブ / 2:カンマ (,) / 3:スペース / 4:セミコロン (;) // 5:なし / 6:引数 Delimiterで指定された文字 Type.Missing, // (省略可能)Password Type.Missing, // (省略可能)WriteResPassword Type.Missing, // (省略可能)IgnoreReadOnlyRecommended Type.Missing, // (省略可能)Origin Type.Missing, // (省略可能)Delimiter Type.Missing, // (省略可能)Editable Type.Missing, // (省略可能)Notify Type.Missing, // (省略可能)Converter Type.Missing, // (省略可能)AddToMru Type.Missing, // (省略可能)Local Type.Missing // (省略可能)CorruptLoad ); Console.Write("エンターキーでExcelを閉じる: "); Console.ReadLine(); oWorkbook.Close(); oXls.Quit(); // これを忘れてはダメ #pragma warning disable CA1416 // プラットフォームの互換性の検証 _ = Marshal.ReleaseComObject(oWorkbook); _ = Marshal.ReleaseComObject(oXls); #pragma warning restore CA1416 // プラットフォームの互換性の検証 Console.ReadLine(); } } }
参考:
- Excelファイルにアクセスするには?[C#、VB]:.NET TIPS – @IT
- .NET core 3.0 and MS Office Interop – Stack Overflow
- .Net Core でCOMコンポーネントを利用する – Qiita
.NET 5 / .NET CoreでCCWしたいときには以下を参照。
コメント